C# 1018번 체스판 다시 칠하기
2022. 1. 10. 13:32ㆍ코딩테스트 문제 풀이/[백준] 브루트 포스
728x90
반응형
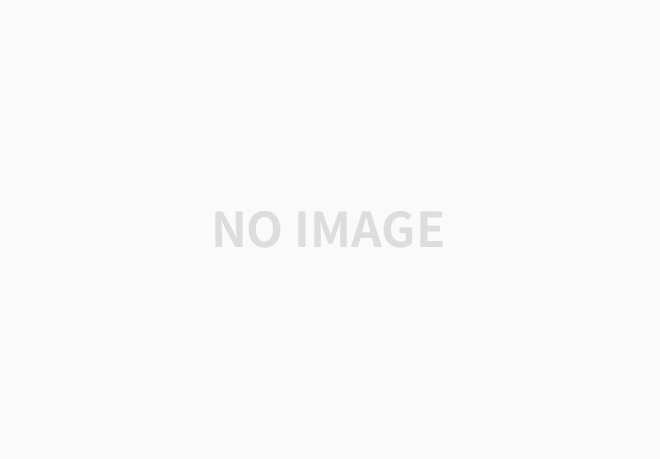
코드 :
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _4
{
class Program
{
static void Main(string[] args)
{
string input = Console.ReadLine();
string[] inputData = input.Split(' ');
int N = Convert.ToInt32(inputData[0]);
int M = Convert.ToInt32(inputData[1]);
string[,] board = new string[N, M];
string[,] Chess = new string[8, 8];
int BlackCount = 0;
int WhiteCount = 0;
int count = 100;
for (int i = 0; i < N; i++) //예제 입력과 동일한 보드판 만들기
{
string BlackAndWrite = Console.ReadLine();
BlackAndWrite.ToCharArray();
for (int j = 0; j < M; j++)
{
board[i, j] = BlackAndWrite[j].ToString();
}
}
for (int i = 0; i < N - 7 ; i++) //좌상단 값의 X인덱스
{
for (int j = 0; j < M - 7 ; j++) //좌상단 값의 y인덱스
{
for (int k = 0; k < 8; k++)
{
for (int l = 0; l < 8; l++)
{
Chess[k, l] = board[k+i, l+j]; //정해진 좌상단부터 시작한 8x8체스판 출력
}
}
BlackCount = ChessCompareToBlack(Chess, i, j); //'B'부터 시작한것으로 변환
WhiteCount = ChessCompareToWhite(Chess, i, j); //'W'부터 시작한것으로 변환
if (BlackCount >= WhiteCount) //변환된 체스판중 어느것이 더 변화가 적었나를 확인
{
if (count >= WhiteCount) //전체 중 어느것이 더 적은 변화인가 확인
{
count = WhiteCount;
}
}
else
{
if (count >= BlackCount) //전체 중 어느것이 더 적은 변화인가 확인
count = BlackCount;
}
}
}
Console.WriteLine(count);
}
//좌상단이 검은색부터 시작하는 체스판
public static int ChessCompareToBlack(string[,] Chess, int x, int y)
{
string[,] tmp = new string[8, 8];
for (int i = 0; i < 8; i++)
{
for (int j = 0; j < 8; j++)
{
tmp[i, j] = Chess[i, j];
}
}
string[] black = { "B", "W", "B", "W", "B", "W", "B", "W" };
string[] white = { "W", "B", "W", "B", "W", "B", "W", "B" };
int index = 0;
int BlackCount = 0;
for (int i = 0; i < 8; i++)
{
int k = 0;
for (int j =0; j < 8; j++)
{
if (index == 0)
{
if (tmp[i, j] != black[k])
{
tmp[i, j] = black[k];
BlackCount += 1;
}
}
else if (index % 2 == 1)
{
if (tmp[i, j] != white[k])
{
tmp[i, j] = white[k];
BlackCount += 1;
}
}
else if(index % 2 == 0)
{
if (tmp[i, j] != black[k])
{
tmp[i, j] = black[k];
BlackCount += 1;
}
}
k += 1;
}
index += 1;
}
return BlackCount;
}
//좌상단이 하얀색부터 시작하는 체스판
public static int ChessCompareToWhite(string[,] Chess, int x, int y)
{
string[,] tmp = new string[8,8];
for (int i = 0; i < 8; i++)
{
for (int j = 0; j < 8; j++)
{
tmp[i, j] = Chess[i, j];
}
}
string[] black = { "B", "W", "B", "W", "B", "W", "B", "W" };
string[] white = { "W", "B", "W", "B", "W", "B", "W", "B" };
int index = 0;
int WhiteCount = 0;
for (int i = 0; i < 8; i++)
{
int k = 0;
for (int j = 0; j < 8; j++)
{
if (index == 0)
{
if (tmp[i, j] != white[k])
{
tmp[i, j] = white[k];
WhiteCount += 1;
}
}
else if (index % 2 == 1)
{
if (tmp[i, j] != black[k])
{
tmp[i, j] = black[k];
WhiteCount += 1;
}
}
else if (index % 2 == 0)
{
if (tmp[i, j] != white[k])
{
tmp[i, j] = white[k];
WhiteCount += 1;
}
}
k += 1;
}
index += 1;
}
return WhiteCount;
}
}
}
코드 풀이 :
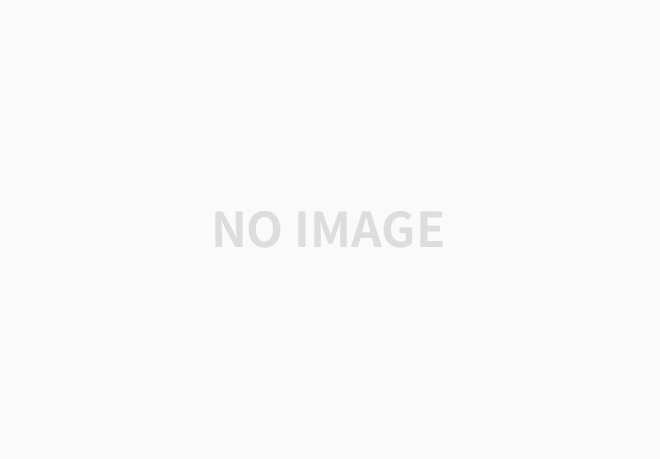
즉 전체보드판에서 체스판으로 만들 일부분을 잘랐을때 좌상단[0,0]값부터 우로, 아래로 8칸이 있어야 하므로 FOR문에서 N-7로 한정지어 준다.
이후 결과로 나올 수 있는 2가지의 형태로 모두 변형시킨후 변형되는 인덱스가 색이 있을때마다 COUNT를 해준다.
이후 더 적은 수로 출력하고 이를 모든 체스판에 반복한다.
깃허브 :
https://github.com/CheongHo-Lee/Algorithm-Study
GitHub - CheongHo-Lee/Algorithm-Study: Algorithm Study For Coding Test
Algorithm Study For Coding Test. Contribute to CheongHo-Lee/Algorithm-Study development by creating an account on GitHub.
github.com
728x90
반응형
'코딩테스트 문제 풀이 > [백준] 브루트 포스' 카테고리의 다른 글
C# 1436번 영화감독 숌 (0) | 2022.01.10 |
---|---|
C# 7568번 덩치 (0) | 2022.01.10 |
C# 2231번 분해합 (0) | 2022.01.10 |
C# 2798번 블랙잭 (0) | 2022.01.10 |