C# 15650번 N과 M(2)
2022. 1. 17. 16:41ㆍ코딩테스트 문제 풀이/[백준] 백트래킹
728x90
반응형
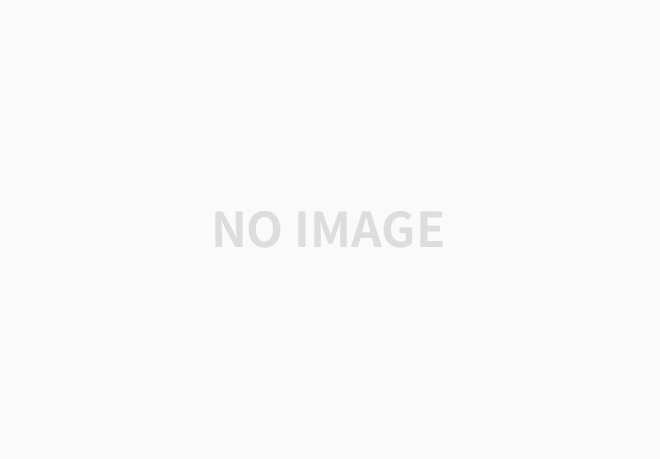
using System;
using System.Text;
namespace beakjoon
{
class _2
{
static int range, length;
// 값 출력을 위한 정수 배열
static int[] arr = new int[9];
// 배열이 오름차순인지 확인하기 위한 배열
static int[] arrSort = new int[9];
// 중복 제거를 위한 bool 배열
static bool[] visited = new bool[9];
// 한번이라도 쓴적이 있는지를 확인하기 위한 bool 배열
static bool[] isUsed = new bool[9];
//DFS 알고리즘
public static void DFS(int cnt)
{
// 출력할 배열이 완성되면 문자열 출력
if (cnt == length)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < length; i++)
{
sb.Append($"{arr[i]} ");
}
Console.WriteLine(sb);
}
// 문자열 완성이 안됬을 시 반복
else
{
for (int i = 1; i <= range; i++)
{
if (!visited[i])
{
if ((cnt > 0 && arr[cnt - 1] < i) || cnt == 0)
{
visited[i] = true;
arr[cnt] = i;
}
else
{
continue;
}
// 재귀 호출
DFS(cnt + 1);
// 다음 문자열 생성을 위해 bool 초기화
visited[i] = false;
}
}
}
}
public static void Main(string[] args)
{
string temp = Console.ReadLine();
string[] read = temp.Split(' ');
//수열의 범위
range = Int32.Parse(read[0]);
//문자열의 길이
length = Int32.Parse(read[1]);
DFS(0);
}
}
}
코드 설명 :
기본적인 틀은 N과M(1)과 같다. 기본코드에 대한 정확한 설명은 아래 링크를 참고하면 좋을거 같다.
C# 15649 N과 M(1)
코드 : using System; using System.Text; namespace beakjoon { class _2 { static int range, length; // 값 출력을 위한 정수 배열 static int[] arr = new int[9]; // 배열이 오름차순인지 확인하기 위한 배..
chlee200530.tistory.com
여기서 오름차순으로 올라가는 수만 출력되도록하는 조건만 추가하면 된다.
if ((cnt > 0 && arr[cnt - 1] < i) || cnt == 0)
{
visited[i] = true;
arr[cnt] = i;
}
깃허브 :
GitHub - CheongHo-Lee/Algorithm-Study: Algorithm Study For Coding Test
Algorithm Study For Coding Test. Contribute to CheongHo-Lee/Algorithm-Study development by creating an account on GitHub.
github.com
728x90
반응형
'코딩테스트 문제 풀이 > [백준] 백트래킹' 카테고리의 다른 글
C# 14888번 연산자 끼워넣기 (0) | 2022.01.17 |
---|---|
C# 9663번 N-Queen (0) | 2022.01.17 |
C# 15652번 N과 M(4) (0) | 2022.01.17 |
C# 15651번 N과 M(3) (0) | 2022.01.17 |
C# 15649번 N과 M(1) (0) | 2022.01.17 |